Customize Social Share Buttons
Bit of Flavor to Induce User Interaction
The default sharing buttons from the major social networking platforms look like this:

It has the following flaws to embed these buttons to websites.
- Difficult for everyone but developers
- Slows down the speed of loading websites
- Same presentation for everyone, every company
The hands-on developers resolve the problems #1. Some clients or project managers wouldn’t realize sharing buttons are making websites slow. So developers decide to pretend this is not happening.
What most of your clients and manager argue would be “Can we change the design?”
Yes, we can.
URLs for Sharing
The hardest part of customizing sharing buttons is to find proper URLs on each social platforms. I’ve done the job for you. Here is a list of URLs for sharing on major platforms. Click the names of platforms that are links in the table; you can jump to their documentations.
Platform | URL |
---|---|
https://www.facebook.com/sharer/sharer.php?u=[URL] | |
Facebook Messenger | https://www.facebook.com/dialog/send?app_id=[APP_ID]&redirect_uri=https%3A%2F%2Fwww.facebook.com&link=[URL] |
https://twitter.com/intent/tweet?url=[URL] | |
https://www.pinterest.com/pin/create/button/?&url=[URL] | |
https://www.linkedin.com/sharing/share-offsite/?url=[URL] | |
https://www.reddit.com/submit?url=[URL] | |
Tumblr | https://www.tumblr.com/widgets/share/tool?posttype=link&canonicalUrl=[URL] |
https://api.whatsapp.com/send?text=[URL] | |
https://getpocket.com/edit?url= |
To open a Messenger’s Send page, you need your website registered on their Developer Dashboard and to get the ID.
Replace [URL] with the URL you want to share. For example, if you want to share the home page of this site on Facebook, type https://www.facebook.com/sharer/sharer.php?u=https%3A%2F%2Fwww.zacfukuda.com%2F in the URL bar of your browser and hit enter. You will see the page shown below—I assume you are logged in to Facebook.
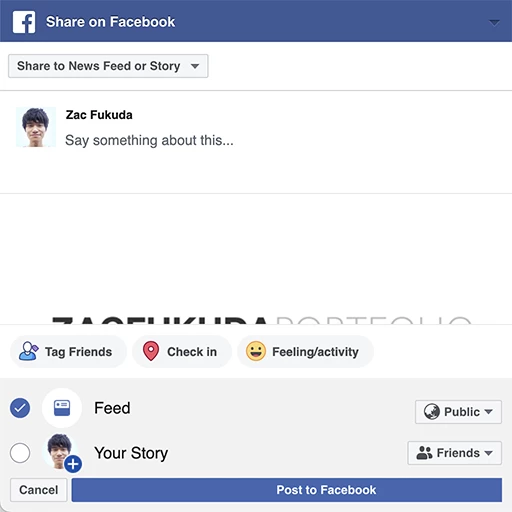
In Japan
Since I live in Japan and need references to Japanese companies, I keep a note of URLs of those companies.
Platform | URL |
---|---|
Line | https://social-plugins.line.me/lineit/share?url=[URL] |
Hatena Bookmark | https://b.hatena.ne.jp/entry/panel/?url=[URL] |
Version1: Pure HTML
Once you know the URLs for sharing, the easiest way to customize sharing buttons is to set those URLs as href of <a> tags. The HTML would be like as follows for Facebook and Twitter:
<a href="https://www.facebook.com/sharer/sharer.php?u=https%3A%2F%2Fwww.zacfukuda.com%2F" target="_blank">Share</a>
<a href="https://twitter.com/intent/tweet?url=https%3A%2F%2Fwww.zacfukuda.com%2F" target="_blank">Tweet</a>
Note that the inside of <a> tag can be any markup. You don’t even need JavaScript.
If you make the images of icons of those two platforms and replace the text with <img> tag in the example above, you will probably get what you want for visual presentation.
Pure HTML solution satisfies the minimum requirements for customization. But let’s make it more user friendly.
Version 2: Dialog with JavaScript
The default sharing buttons open a new dialog-like window when clicked. We imitate this behavior using window.open() API.
Most of sharing occur for the current page viewed. We get the URL of the current page with location.href, append the value to the URL of platforms, and use this combined value for window.open().
The example HTML and JavaScript are shown below:
HTML
<a class="share-button" href="https://www.facebook.com/sharer/sharer.php?u=">facebook</a>
<a class="share-button" href="https://twitter.com/intent/tweet?url=">tweet</a>
JavaScript
document.querySelectorAll('.share-button').forEach(btn => {
btn.addEventListener('click', (e) => {
e.preventDefault()
let w = Math.min(640, screen.width - 32),
h = Math.min(480, screen.height - 32),
l = (screen.width - w) / 2,
t = (screen.height - h) / 2
window.open(btn.href + location.href, '_blank', `width=${w},height=${h},left=${l},top=${t}`)
})
})
Version 3: Polish
After getting the result that you want, what we need to do as a good developer is to polish code, making it more reusable, extendable, and maintainable.
Here is a list of modifications I want to add to the previous code:
- JavaScript object which contains the URLs of majors platforms for sharing
- Aliases, like fb for Facebook, tw for Twitter
- data-platform attribute to the anchor; use this value to get a proper sharing URLs
- Global window features for window.open()
- An abstract ShareButton class
- If href is set, use this for sharing; if not, use location.href, i.e. the current page URL
The end code is:
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Custom Share Button</title>
</head>
<body>
<a class="share-button" data-platform="fb">facebook</a>
<a class="share-button" data-platform="tw">tweet</a>
<a class="share-button" data-platform="pin">pinterest</a>
<a class="share-button" data-platform="in">linkedin</a>
<a class="share-button" data-platform="reddit">reddit</a>
<a class="share-button" data-platform="tumblr">tumblr</a>
<a class="share-button" data-platform="whatsapp">whatsapp</a>
<a class="share-button" data-platform="pocket">pocket</a>
<a class="share-button" data-platform="messenger">messenger</a>
<script src="ShareButton.js"></script>
<script>document.querySelectorAll('.share-button').forEach(node => new ShareButton(node))</script>
</body>
</html>
ShareButton.js
var sharePlatforms = {
facebook: 'https://www.facebook.com/sharer/sharer.php?u=',
twitter: 'https://twitter.com/intent/tweet?url=',
pinterest: 'https://www.pinterest.com/pin/create/button/?&url=',
linkedin: 'https://www.linkedin.com/sharing/share-offsite/?url=',
reddit: 'https://www.reddit.com/submit?url=',
tumblr: 'https://www.tumblr.com/widgets/share/tool?posttype=link&canonicalUrl=',
whatsapp: 'https://api.whatsapp.com/send?text=',
pocket: 'https://getpocket.com/edit?url=',
messenger: 'https://www.facebook.com/dialog/send?app_id=1536799263313447&redirect_uri=https%3A%2F%2Fwww.facebook.com&link=',
line: 'https://social-plugins.line.me/lineit/share?url=',
hatena: 'https://b.hatena.ne.jp/entry/panel/?url=',
}
sharePlatforms.fb = sharePlatforms.facebook
sharePlatforms.tw = sharePlatforms.twitter
sharePlatforms.pin = sharePlatforms.pinterest
sharePlatforms.in = sharePlatforms.linkedin
var shareWindowFeatures = (function() {
let w = Math.min(640, screen.width - 32),
h = Math.min(480, screen.height - 32),
l = (screen.width - w) / 2,
t = (screen.height - h) / 2
return `width=${w},height=${h},left=${l},top=${t}`
})()
class ShareButton {
constructor(node) {
if (!node || !sharePlatforms[node.dataset.platform]) return
this.url = sharePlatforms[node.dataset.platform]
this.href = node.href ? encodeURI(node.href) : location.href
node.onclick = this.share.bind(this)
}
share(e) {
e.preventDefault()
window.open(this.url + this.href, '_blank', shareWindowFeatures)
}
}
If you want to add other platforms not listed in the code above, find their sharing URL and add key/value to sharePlatforms.
Third Parties
I love hard coding; I love making my own. That is one of reasons why I work in this industry. Nevertheless, time is important and precious. It might be wasting time to make something that is already existed. I see your point.
So, here are some third parties to implement custom sharing buttons.
AddToAny
No registration required, covers many world-wide platforms, has plugins for major CMS including WordPress. The downside of AddToAny is that you cannot use your own custom buttons but ones they prepare for you. (This fact led me to make and code my own buttons.)
FYI, the buttons of AddToAny look like this:

AddThis
Registration required. I haven’t used AddThis before, so I cannot state anything honest. It has many options that you could use as a marketing strategy like floating bar, and pop-up.
ShareThis
Registration required. I haven’t used ShareThis either, so I cannot state anything honest again. It seems that while AddThis focuses on visual presentation and integration, ShareThis focuses on data analyzation and globalization.
Download
Please download the source code at here.